A Guide to Converting React Markdown to HTML
Learn how to convert Markdown to HTML in React apps using react-markdown and remark libraries. This guide covers basic and advanced usage, including custom components and integrations with other libraries.
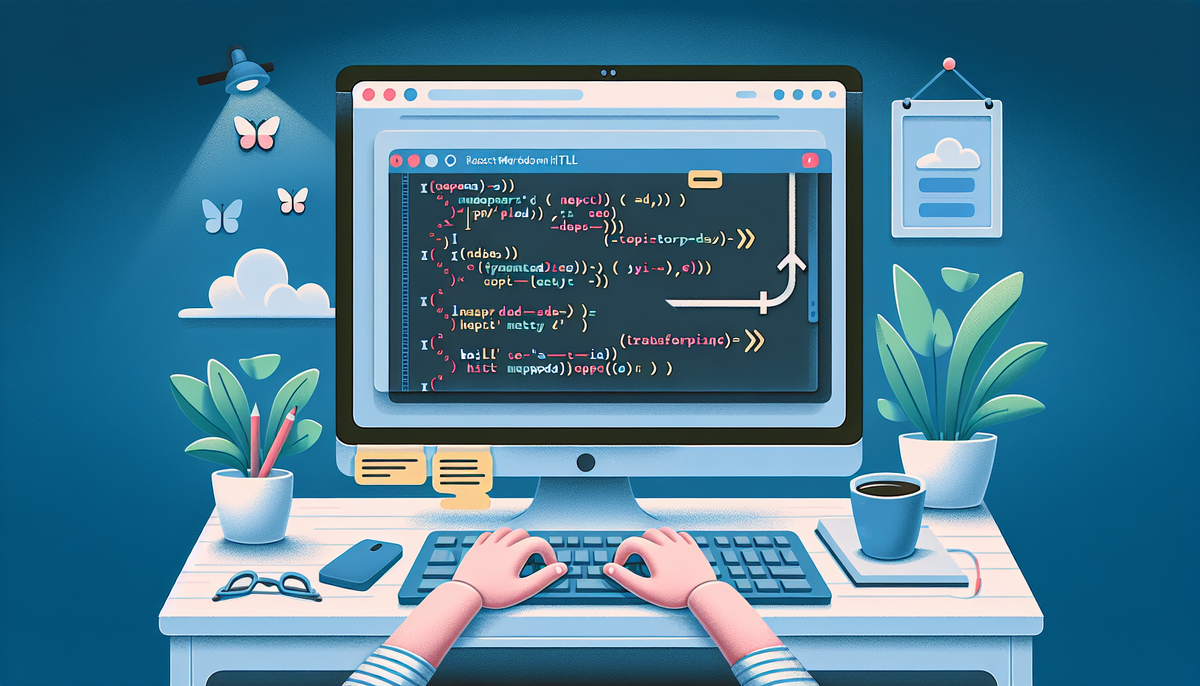
"Explore our suite of free Markdown toolsto convert, format, and enhance your documents with ease."
In modern web development, Markdown has become a popular markup language. Whether you're writing documentation, blog posts, or README files, Markdown stands out for its simplicity and readability. Converting Markdown to HTML in a React application is a common requirement. This article will detail how to achieve this conversion in a React app.
What is Markdown?
Markdown is a lightweight markup language that uses plain text formatting. It can be converted to HTML or other formats. Its simple syntax requires little to no learning curve, which is why developers love it.
Why Use Markdown in React?
- Simplicity: Markdown's syntax is straightforward and easy to read and write.
- Flexibility: It can be easily embedded within React components, enhancing code maintainability.
- User-Friendly: Suitable for collaboration with non-technical team members who can produce content easily.
How to Convert Markdown to HTML?
In React, there are several libraries you can use to convert Markdown to HTML, with remark
and react-markdown
being the most common. Let's dive into how to use these libraries.
Installing Dependencies
First, install remark
and react-markdown
in your project:
npm install react-markdown remark-gfm
Basic Usage
Below is a basic React component that converts Markdown to HTML and renders it on the page:
import React from 'react';
import ReactMarkdown from 'react-markdown';
import remarkGfm from 'remark-gfm';
const markdown = `
# Title
This is some text.
`;
const MarkdownToHtml = () => {
return (
<div>
<ReactMarkdown remarkPlugins={[remarkGfm]} children={markdown} />
</div>
);
}
export default MarkdownToHtml;
In this example, we first import the ReactMarkdown
component and the remarkGfm
plugin. Then, we create a Markdown string. Using the ReactMarkdown
component and the remarkPlugins
property, we convert the Markdown string to HTML and render it on the page.
Advanced Usage
Custom Components
You can enhance Markdown rendering by using custom components. For example, using custom code blocks and image components:
import React from 'react';
import ReactMarkdown from 'react-markdown';
import remarkGfm from 'remark-gfm';
import { Prism as SyntaxHighlighter } from 'react-syntax-highlighter';
import { vscDarkPlus } from 'react-syntax-highlighter/dist/esm/styles/prism';
const markdown = `
# Example Code
\`\`\`javascript
console.log('Hello, world!');
\`\`\`
`;
const MarkdownToHtml = () => {
return (
<div>
<ReactMarkdown
children={markdown}
remarkPlugins={[remarkGfm]}
components={{
code({ node, inline, className, children, ...props }) {
const match = /language-(\w+)/.exec(className || '');
return !inline && match ? (
<SyntaxHighlighter style={vscDarkPlus} language={match[1]} PreTag="div" {...props}>
{String(children).replace(/\n$/, '')}
</SyntaxHighlighter>
) : (
<code className={className} {...props}>
{children}
</code>
);
}
}}
/>
</div>
);
}
export default MarkdownToHtml;
In this example, we included the react-syntax-highlighter
library to support syntax highlighting for code blocks. We customized the code
component to use SyntaxHighlighter
for rendering code blocks.
Integrating with Other Libraries
You can also integrate Markdown with other libraries, such as highlight.js
for code highlighting or katex
for math formula rendering.
import React from 'react';
import ReactMarkdown from 'react-markdown';
import rehypeKatex from 'rehype-katex';
import remarkMath from 'remark-math';
import 'katex/dist/katex.min.css';
const markdown = `
# Math Formula
$$
E = mc^2
$$
`;
const MarkdownToHtml = () => {
return (
<div>
<ReactMarkdown
children={markdown}
remarkPlugins={[remarkMath]}
rehypePlugins={[rehypeKatex]}
/>
</div>
);
}
export default MarkdownToHtml;
In this example, we use the remark-math
and rehype-katex
plugins to render mathematical formulas in Markdown.
Conclusion
Converting Markdown to HTML and rendering it in a React application is a useful skill. With react-markdown
, remark
, and other plugins, you can accomplish this quickly and efficiently. I hope this article helps you better handle Markdown content in your React projects.
Comments ()