Building a Modern Markdown Editor with React
This article introduces how to use React to build a modern Markdown editor with core features such as real-time preview, syntax highlighting, and advanced editing capabilities. By leveraging a component-based structure and third-party libraries, development becomes more efficient and maintainable.
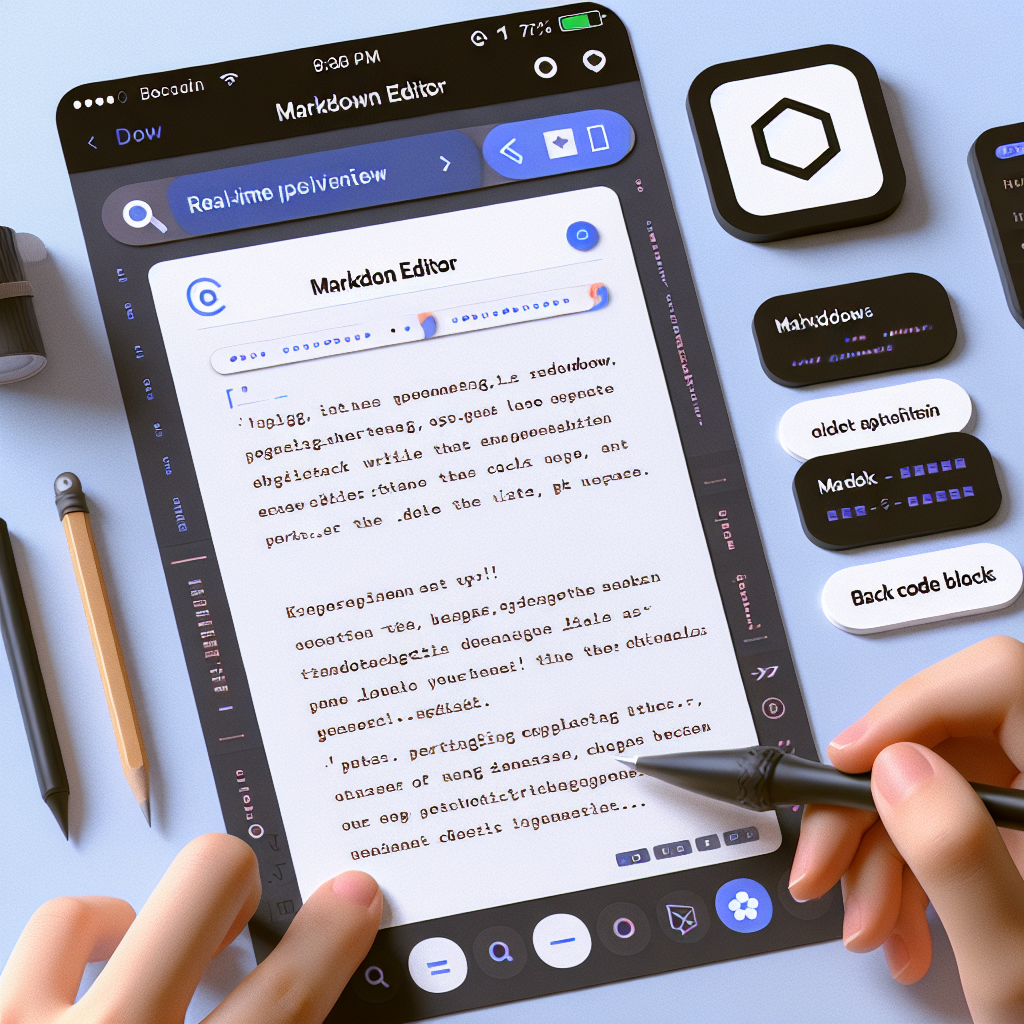
As the complexity of web applications continues to increase, the demand for user-friendly document editing tools becomes more evident. Markdown, as a lightweight markup language, has become a mainstream format for content creation, such as technical documentation and blogs. To provide a seamless Markdown editing experience for users, developing a Markdown editor based on React is an ideal solution. In this article, we will explore how to build a modern Markdown editor using React.
Why Choose React?
React is a popular JavaScript library developed by Facebook for building user interfaces, especially single-page applications. Its component-based and declarative programming style makes developing complex UIs simple and efficient, making React an excellent choice for building dynamic applications like a Markdown editor.
1. Component-Based Structure
React's component system allows developers to break down an application into independent, reusable pieces. For a Markdown editor, this means you can design various parts of the editor—such as the toolbar, preview area, and text input area—as independent components, making maintenance and iteration more convenient.
2. Declarative UI
React expresses UI states and changes through declarative code. This means developers can more intuitively understand how user interactions affect the application's interface state without manual DOM manipulation, making the development process more efficient and readable.
Key Features of a Markdown Editor
When designing a fully functional Markdown editor, consider the following core features:
1. Real-Time Preview
Real-time preview is a major highlight of Markdown editors. As users write Markdown syntax, they should be able to immediately see the formatted results. React's lifecycle methods or Hooks can be used to easily implement this feature for instantaneous preview updates.
2. Syntax Highlighting
Syntax highlighting improves the readability of Markdown files. By choosing an appropriate highlighting library (such as highlight.js
) and integrating it with React, you can add syntax highlighting capabilities to the editor to enhance the user experience.
3. Advanced Editing Features
Features such as auto-completion, keyboard shortcut support, and theme switching can offer users a more advanced and personalized experience. React's rich ecosystem provides numerous third-party libraries and components that can be used to add these features.
Using Third-Party Libraries
When building a Markdown editor, using third-party libraries can greatly speed up the development process. For example, remarkable
or markdown-it
can be used to convert Markdown to HTML, while CodeMirror
or Monaco Editor
can provide powerful text editing capabilities.
Example Code
Below is a simple example code showing how to integrate a basic Markdown editor in React:
import React, { useState } from 'react';
import MarkdownIt from 'markdown-it';
const mdParser = new MarkdownIt();
const MarkdownEditor = () => {
const [content, setContent] = useState('');
const handleChange = (e) => {
setContent(e.target.value);
};
return (
<div className="editor-container">
<textarea
value={content}
onChange={handleChange}
placeholder="Type your markdown here..."
/>
<div
className="preview"
dangerouslySetInnerHTML={{ __html: mdParser.render(content) }}
/>
</div>
);
};
export default MarkdownEditor;
Conclusion
Using React to build a modern Markdown editor allows you to take full advantage of its component-based and declarative benefits, making the development process more efficient and maintainable. By integrating third-party libraries and implementing core features, you can provide users with a responsive and feature-rich document editing experience. Whether for technical document writing or personal blog creation, a React Markdown editor is a tool worth exploring.
Comments ()