Converting Markdown to HTML with Node.js
Learn to convert Markdown to HTML with Node.js using the `marked` library. Install `marked` via npm, write a Node.js script, and customize rendering for enhanced web content display.
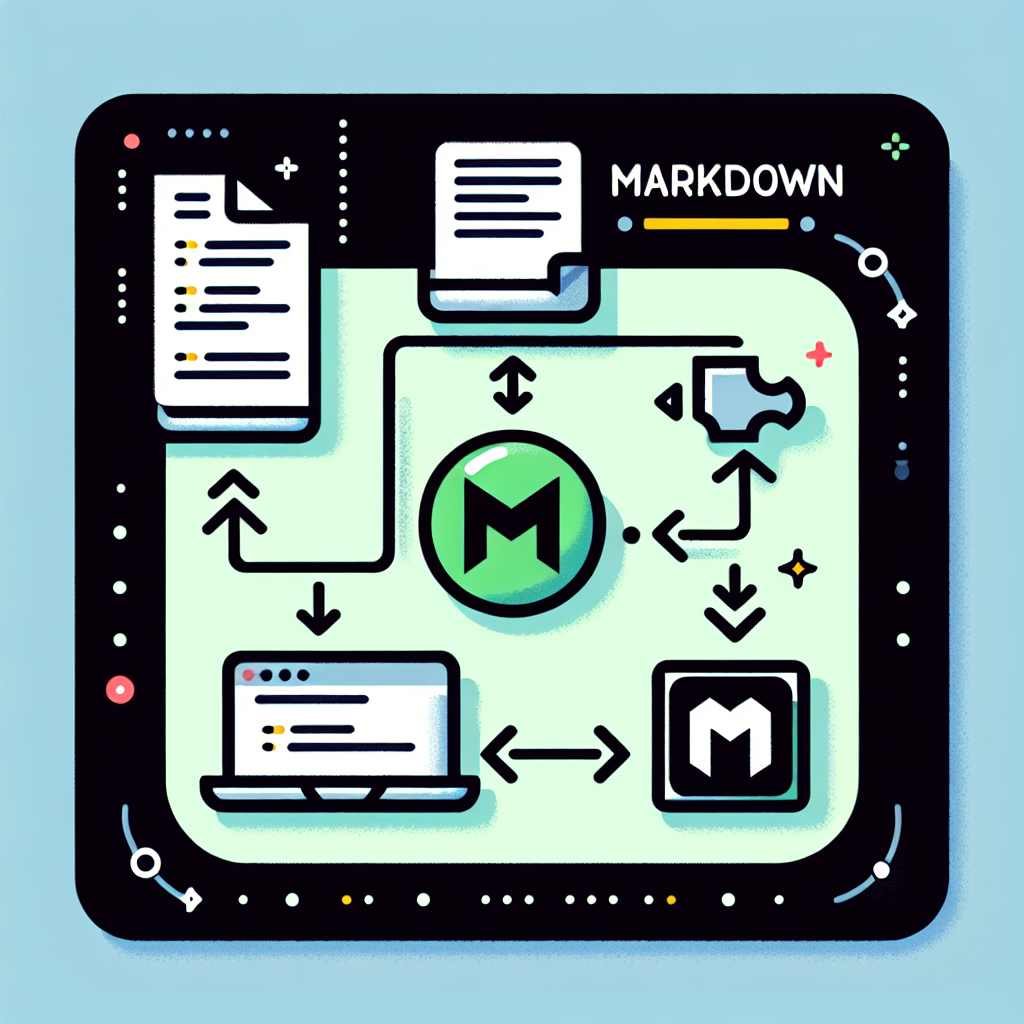
"Tired of manually formatting your Markdown? Try our free, one-click Markdown converter and simplify your writing workflow today!"
In modern web development, Markdown has become a highly popular lightweight markup language. Its concise syntax makes content writing extremely efficient. However, most web applications require converting Markdown content into HTML for display in browsers. This article will guide you through the process of achieving this conversion using Node.js.
Why Choose Node.js?
Node.js is a JavaScript runtime environment based on the Chrome V8 engine, allowing JavaScript to run on the server side. Node.js's non-blocking I/O model and rich ecosystem make it an ideal choice for handling such tasks.
Installing Necessary Dependencies
First, we need to install some essential npm packages to assist us in converting Markdown to HTML. We will use the popular marked
Markdown parser.
npm install marked
Writing the Conversion Script
Next, we will write a simple Node.js script to read a Markdown file and convert it to HTML.
- Create a New File: In your project directory, create a file named
convert.js
. - Write the Script: In the
convert.js
file, write the following code:
const fs = require('fs');
const marked = require('marked');
// Read the Markdown file
fs.readFile('input.md', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
// Convert Markdown to HTML
const html = marked(data);
// Write the HTML to a file
fs.writeFile('output.html', html, (err) => {
if (err) {
console.error('Error writing file:', err);
return;
}
console.log('Conversion complete!');
});
});
- Create a Markdown File: In your project directory, create a file named
input.md
and add some Markdown content.
# Hello World
This is a simple Markdown file.
- Item 1
- Item 2
- Item 3
- Run the Script: Execute the script in the terminal with the following command:
node convert.js
If everything goes smoothly, you will see a file named output.html
in your project directory containing the converted HTML content.
Customizing Markdown Rendering
The marked
library allows us to customize the rendering of Markdown. For example, we can add code highlighting or modify the default HTML output.
const marked = require('marked');
const hljs = require('highlight.js');
// Custom renderer
const renderer = new marked.Renderer();
renderer.code = (code, language) => {
const validLanguage = hljs.getLanguage(language) ? language : 'plaintext';
return `<pre><code class="hljs ${validLanguage}">${hljs.highlight(validLanguage, code).value}</code></pre>`;
};
marked.setOptions({
renderer: renderer,
highlight: (code, language) => {
const validLanguage = hljs.getLanguage(language) ? language : 'plaintext';
return hljs.highlight(validLanguage, code).value;
}
});
// Read the Markdown file and convert to HTML
fs.readFile('input.md', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
const html = marked(data);
fs.writeFile('output.html', html, (err) => {
if (err) {
console.error('Error writing file:', err);
return;
}
console.log('Conversion complete!');
});
});
In this example, we use highlight.js
to add syntax highlighting to code blocks.
Conclusion
By using Node.js and the marked
library, we can easily convert Markdown content into HTML. This not only improves the efficiency of content writing but also makes the display of content in web applications more flexible and aesthetically pleasing. I hope this article has been helpful to you. Good luck with your Node.js development!
Comments ()