Converting Markdown to PDF with Python: A One-Stop Guide
This article briefly outlines the method of converting Markdown to PDF using Python and the `markdown`, `pdfkit` libraries, including custom styling and handling images and links.
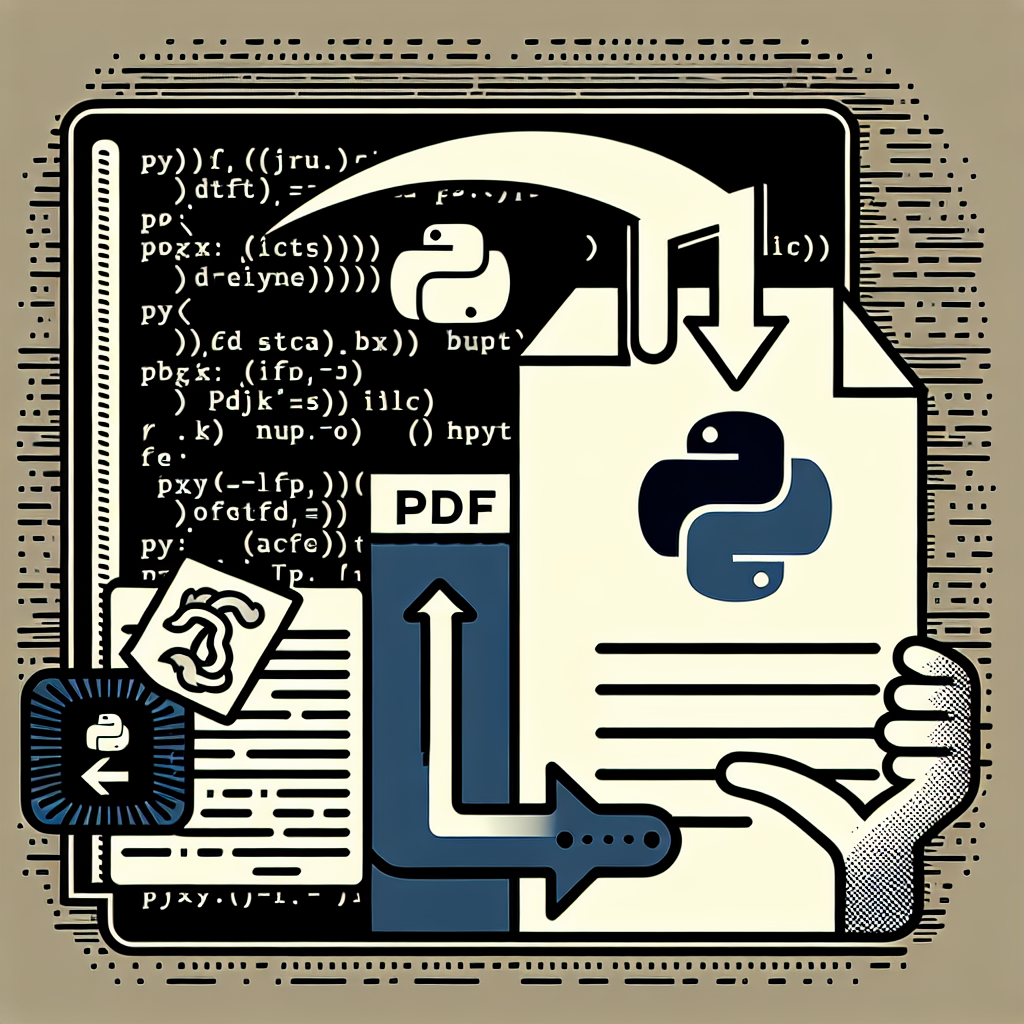
"Don't waste another minute formatting Markdown by hand. Try our free tools now and see the difference!"
In today's digital age, document format conversion has become a part of daily work. Particularly, converting Markdown files to PDF format is very common in technical writing and document management. Python, as a powerful programming language, provides various libraries and tools to accomplish this task. This article will detail how to use Python to convert Markdown files to PDF and provide some practical code examples.
1. Prerequisites
Before you start, ensure that Python is installed on your system. If not, you can download and install it from the Python official website.
Additionally, we need to install some Python libraries to help us complete the conversion task. The main libraries used include:
markdown
: For parsing Markdown text.pdfkit
: For generating PDF files.wkhtmltopdf
: A dependency ofpdfkit
, used to convert HTML to PDF.
You can install these libraries using the following command:
pip install markdown pdfkit
Also, you need to download and install wkhtmltopdf
from the wkhtmltopdf official website.
2. Parsing Markdown Text with the markdown
Library
First, we need to parse the Markdown text into HTML format. The markdown
library provides a simple and easy-to-use API to accomplish this task.
import markdown
def markdown_to_html(markdown_text):
html = markdown.markdown(markdown_text)
return html
3. Generating PDF Files with the pdfkit
Library
Next, we will use the pdfkit
library to convert the HTML text to a PDF file. The pdfkit
library relies on wkhtmltopdf
, so ensure you have correctly installed wkhtmltopdf
.
import pdfkit
def html_to_pdf(html, output_path):
pdfkit.from_string(html, output_path)
4. Complete Example
Now, we will combine the above steps to create a complete script to convert a Markdown file to a PDF file.
import markdown
import pdfkit
def markdown_to_html(markdown_text):
html = markdown.markdown(markdown_text)
return html
def html_to_pdf(html, output_path):
pdfkit.from_string(html, output_path)
def markdown_to_pdf(markdown_path, pdf_path):
with open(markdown_path, 'r', encoding='utf-8') as file:
markdown_text = file.read()
html = markdown_to_html(markdown_text)
html_to_pdf(html, pdf_path)
if __name__ == "__main__":
markdown_path = 'example.md'
pdf_path = 'output.pdf'
markdown_to_pdf(markdown_path, pdf_path)
5. Advanced Features
5.1 Custom Styles
You can customize the appearance of the generated PDF file by adding CSS styles. Just include the CSS styles in the HTML text.
def markdown_to_html(markdown_text):
css = """
<style>
body {
font-family: Arial, sans-serif;
}
h1 {
color: #333333;
}
</style>
"""
html = markdown.markdown(markdown_text)
return css + html
5.2 Handling Images and Links
By default, pdfkit
can handle images and links in Markdown. Ensure the image paths are correct and the links are accessible.
6. Conclusion
By using Python and the relevant libraries, we can easily convert Markdown files to PDF format. This article has introduced how to use the markdown
and pdfkit
libraries to accomplish this task and provided some practical code examples. We hope this article is helpful to you, making you more proficient in document format conversion.
Comments ()