Creating Elegant Content Displays with SwiftUI and Markdown
SwiftUI's powerful UI tools and Markdown's simple text formatting enable elegant content displays. Use native support or third-party libraries to handle Markdown flexibly and customize styles, enhancing the user reading experience.
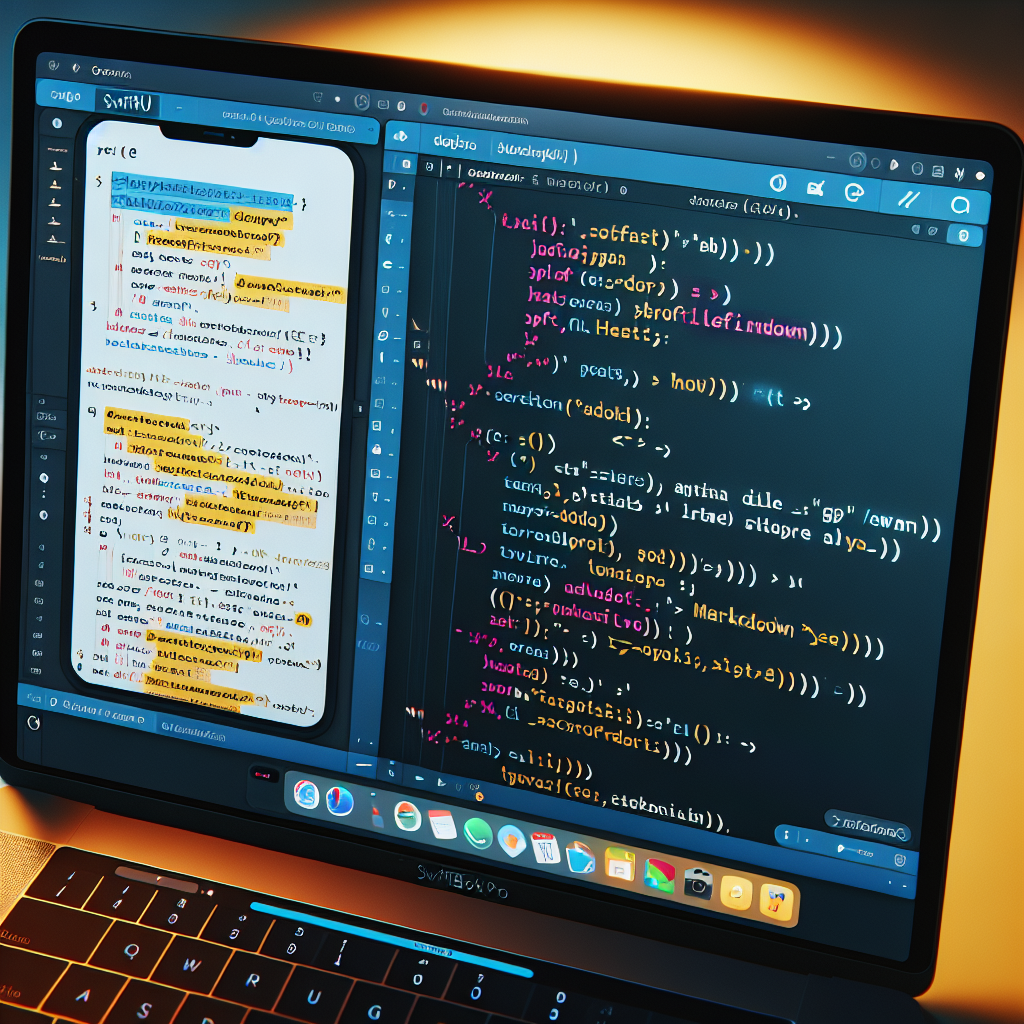
"Need to convert or format Markdown? Check out our free tools– they're easy to use and always available."
SwiftUI offers developers a powerful and flexible tool for building user interfaces, and its concise declarative syntax has made it a popular choice in iOS development. Meanwhile, Markdown, a lightweight markup language, simplifies writing and formatting text. Combining SwiftUI and Markdown allows you to create elegant and feature-rich content displays effortlessly. This article will explore how to effectively use Markdown within SwiftUI.
What is Markdown?
Markdown is a lightweight markup language that uses an easily readable and writable plain text format syntax, which can quickly be converted to HTML. Its simplicity and readability have made Markdown a popular choice for writing documentation, blogs, and other text content.
Combining SwiftUI and Markdown
In SwiftUI, the Text
view is the primary component for displaying text. With iOS 15 and macOS 12 and later, SwiftUI added native support for Markdown, simplifying the process of formatting text using Markdown.
Using Markdown in SwiftUI
To use Markdown-formatted text in a SwiftUI app, simply pass the Markdown content to the Text
view. Here is a basic example:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("""
# Welcome to SwiftUI
**SwiftUI** is a powerful framework for building UIs.
Here are some features:
- Declarative Syntax
- Hot Reloading
- Integration with Xcode
""")
.padding()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
In the code above, we used Markdown syntax to format the text. When run, you will see a formatted content including a header, bold text, and a list.
Handling Complex Markdown Content
If your app needs to support more complex Markdown content display, consider using a third-party library like SwiftMarkdown. This library provides comprehensive Markdown rendering capabilities and can seamlessly integrate with SwiftUI.
Here is an example of rendering Markdown with the Down library:
import SwiftUI
import Down
struct MarkdownView: UIViewRepresentable {
var markdown: String
func makeUIView(context: Context) -> UIView {
let label = try? DownView(frame: .zero, markdownString: markdown)
return label ?? UIView()
}
func updateUIView(_ uiView: UIView, context: Context) {
if let label = uiView as? DownView {
try? label.update(markdownString: markdown)
}
}
}
struct ContentView: View {
var body: some View {
MarkdownView(markdown: """
# Advanced Markdown Example
**Down** is a robust Markdown renderer for Swift.
`Code blocks` are also supported.
> Block quotes are great for highlighting texts.
- Customizable
- Easy to use
""")
.padding()
}
}
Note: Ensure you integrate the Down library into your project before using the code above.
Customizing Markdown Styles
Sometimes, you might want to customize the display style of Markdown content. This can be achieved by modifying the text style of SwiftUI’s Text
view, such as changing the font, color, etc.:
Text("""
# Custom Styled Markdown
SwiftUI makes it easy to customize text styles.
""")
.font(.largeTitle)
.foregroundColor(.blue)
.bold()
.padding()
By adjusting the modifiers for the Text
view, you can easily create Markdown content displays that align with your app’s style.
Conclusion
The combination of SwiftUI and Markdown provides developers with an efficient and elegant way to display formatted text content. Whether it's simple text display or complex document rendering, SwiftUI can handle it with flexibility. By mastering and leveraging these technologies, you can deliver an outstanding reading experience to your users.
Hopefully, this article has been helpful in guiding you on how to use Markdown within SwiftUI development!
Comments ()