Exploring Newline Handling in React Markdown
This article explores methods to handle newlines in React using React Markdown, offering solutions like double spaces, <br> tags, and custom text renderers, along with best practices to ensure proper Markdown content rendering.
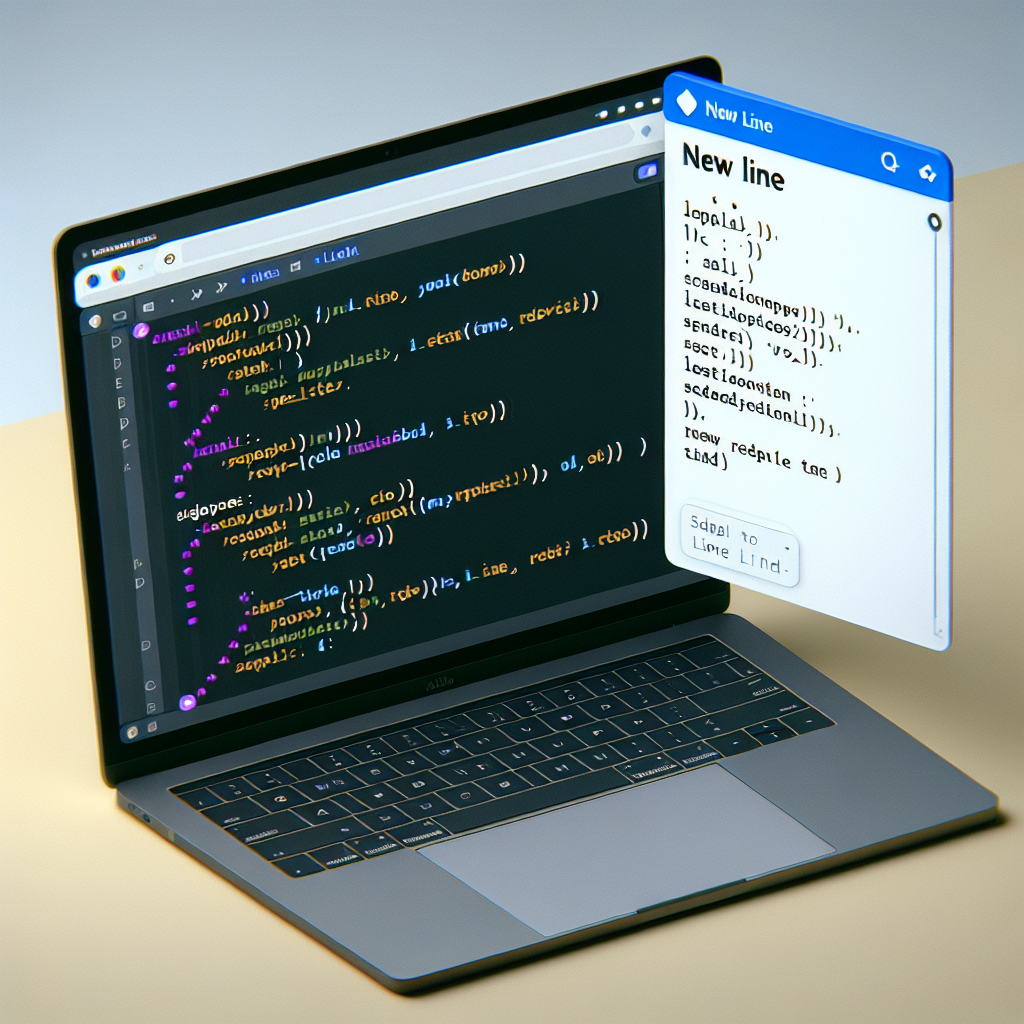
"Why struggle with Markdown formatting? Our free tools make it easy to create beautiful, professional-looking documents in seconds."
When developing with React, handling newlines in Markdown text can sometimes be tricky. React Markdown, a powerful library, effectively renders Markdown content into HTML. This article will explore how to handle newlines in Markdown text within React, providing best practices and code examples.
Introduction to React Markdown
React Markdown is a lightweight React component that allows developers to easily render Markdown content as React elements. With this component, you can efficiently display Markdown content in a React application without manually writing HTML code.
The Newline Issue
In Markdown, single newline characters are typically ignored, requiring two or more newlines for an actual line break. This behavior usually aligns with expectations, but there are cases where we might want every newline character in Markdown to be preserved and rendered as an HTML <br>
.
Solutions
-
Using Double Space for Line Breaks: In Markdown, you can enforce a line break by adding two spaces at the end of a line. For example:
This is the first line This is the second line
-
Using the
<br>
Tag: You can also use the HTML<br>
tag directly in your Markdown for line breaks:This is the first line<br> This is the second line
-
Configuring the React Markdown Component: By configuring the React Markdown component, you can customize the Markdown parser to handle newlines. Here is an implementation example:
First, install
react-markdown
:npm install react-markdown
Then, use it in your React component and handle newlines:
import React from 'react'; import ReactMarkdown from 'react-markdown'; const markdown = ` This is the first line This is the second line `; const renderers = { text: ({ children }) => children.split('\n').map((line, index) => ( <React.Fragment key={index}> {line} {index < children.split('\n').length - 1 && <br />} </React.Fragment> )), }; const MarkdownComponent = () => ( <div> <ReactMarkdown source={markdown} renderers={renderers} /> </div> ); export default MarkdownComponent;
In the code above, we customized the text renderer to transform every newline character into an
<br>
tag.
Best Practices
- Clarify Requirements: Ensure you understand your output goals when using Markdown. Do you need strict Markdown line breaks, or should every newline character be preserved?
- Custom Rendering: Use custom renderers to flexibly handle Markdown according to your needs.
- Test and Adjust: In real projects, ensure to test the rendering of Markdown content and refine the implementation to achieve the best results.
Conclusion
Handling newlines in Markdown content within a React project is not complex. By understanding Markdown rules and configuring the React Markdown component, you can flexibly achieve the desired newline effects. We hope this article helps you better handle newline issues in Markdown within your React projects.
If you need any further modifications or need to add specific information, please let me know!
Comments ()