How to Implement "Copy to Clipboard" Functionality in Markdown
Embed HTML and JavaScript in Markdown to add a "copy to clipboard" button for code blocks. Use CSS to style the button. This improves usability for sharing and referencing content.
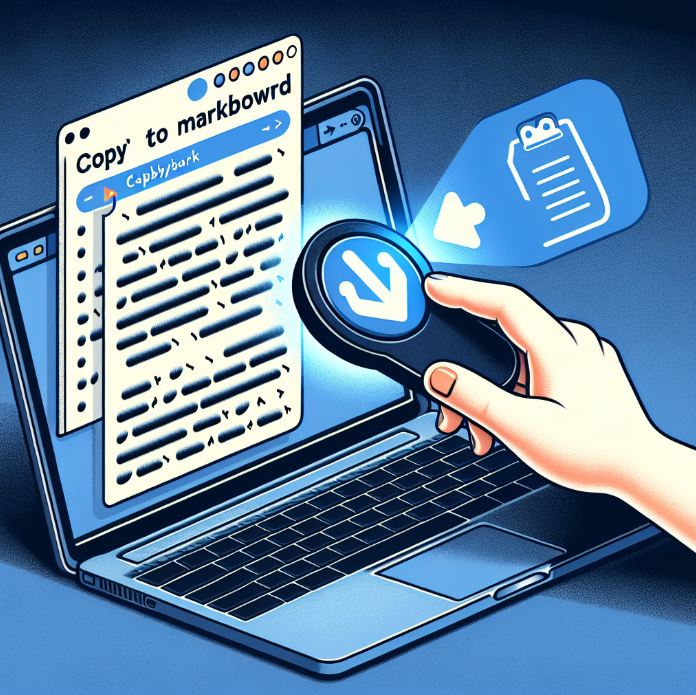
"Tired of manually formatting your Markdown? Try our free, one-click Markdown converter and simplify your writing workflow today!"
When writing and reading Markdown documents, being able to quickly copy text to the clipboard is a highly practical feature. Whether for sharing code snippets or quoting document content, this feature can significantly enhance efficiency. This article will detail how to implement "copy to clipboard" functionality in Markdown.
What is Markdown?
Markdown is a lightweight markup language designed to format text using simple syntax. It can be easily converted to HTML and is suitable for writing documents, blogs, README files, and more. Common Markdown syntax includes:
- Headings: Use
#
, e.g.,# Heading 1
- Bold: Wrap text with
**
, e.g.,**bold text**
- Italic: Wrap text with
*
, e.g.,*italic text*
- Code blocks: Wrap text with triple backticks, e.g.,
code block
- Lists: Use
-
or numbers, e.g.,- List item
Implementing "Copy to Clipboard" Functionality
To implement the "copy to clipboard" functionality in Markdown, we typically need to use JavaScript and some HTML tags. Here are the basic steps and a code example to achieve this.
1. Include Necessary HTML and JavaScript
In Markdown, embedding HTML is feasible. We need to add a button and the relevant JavaScript code to achieve the copy function. Here’s a simple example:
# Example Code Block
Here is a code block that can be copied:
```html
<pre id="code-block">
<code>
function helloWorld() {
console.log("Hello, world!");
}
</code>
</pre>
<button onclick="copyToClipboard()">Copy Code</button>
<script>
function copyToClipboard() {
var codeBlock = document.getElementById('code-block').innerText;
var tempInput = document.createElement('textarea');
tempInput.value = codeBlock;
document.body.appendChild(tempInput);
tempInput.select();
document.execCommand('copy');
document.body.removeChild(tempInput);
alert('Code copied to clipboard');
}
</script>
2. Optimize Button Style
To enhance user experience, you can style the button with simple CSS:
<style>
button {
background-color: #4CAF50;
color: white;
border: none;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
border-radius: 4px;
}
button:hover {
background-color: #45a049;
}
</style>
3. Ensure Consistent Code Block Formatting
To ensure consistent formatting of code blocks, you can use some online Markdown editors or plugins that automatically add the "copy to clipboard" button to code blocks. Examples include:
- Markdown Here: A browser extension that allows you to use Markdown anywhere.
- Typora: A WYSIWYG Markdown editor that supports custom code block styles.
- GitHub: On GitHub's code snippet pages, a "copy" button automatically appears next to code blocks.
Conclusion
By combining HTML and JavaScript, you can easily implement "copy to clipboard" functionality in Markdown documents. This not only enhances the usability and user experience of your documents but also provides great convenience for sharing and quoting content. I hope the examples and steps in this article help you implement this feature in your Markdown projects. If you have more needs or encounter issues, feel free to discuss them in the comments.
Comments ()