Integrating ngx-markdown in an Angular Application
Integrate ngx-markdown in Angular to easily render Markdown content. Install ngx-markdown, configure your module, and use the markdown directive or component to display Markdown files with syntax highlighting.
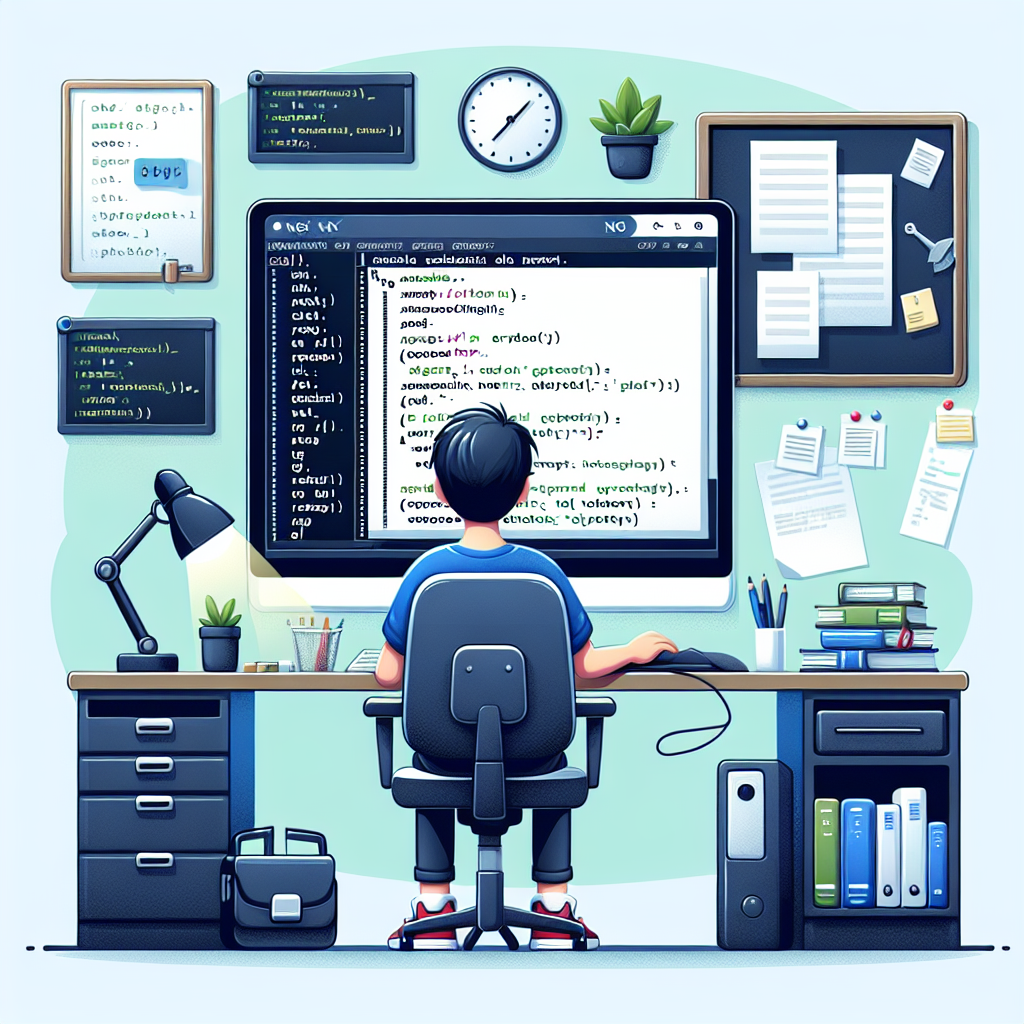
"Don't waste another minute formatting Markdown by hand. Try our free tools now and see the difference!"
Introduction
Angular is a robust framework for building dynamic and client-side web applications. However, when it comes to content creation, Markdown offers a simple and flexible way to write formatted text. Integrating Markdown into an Angular application can streamline content management, especially for blogs, documentation sites, and any content-heavy platform. In this article, we will explore how to utilize ngx-markdown
, a powerful library that enables Markdown support in Angular.
What is ngx-markdown?
ngx-markdown
is an Angular library that leverages the marked
and highlight.js
libraries to provide an efficient way to render Markdown content within Angular applications. It simplifies the process of parsing and displaying Markdown files and supports syntax highlighting for code blocks.
Setting Up an Angular Project
If you haven’t set up your Angular project, you can create one using Angular CLI:
ng new my-angular-markdown-app
cd my-angular-markdown-app
Installing ngx-markdown
To use ngx-markdown
, you need to install the library and its dependencies:
npm install ngx-markdown
npm install marked highlight.js
Next, import the MarkdownModule
into your AppModule
:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { MarkdownModule } from 'ngx-markdown';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
MarkdownModule.forRoot()
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Rendering Markdown in a Component
To render Markdown content within a component, use the markdown
directive or the markdown
component provided by ngx-markdown
. Here’s an example using both approaches:
Using markdown
Directive:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<div markdown>
# Welcome to my Angular App
This is a sample Markdown content rendered in **Angular** using *ngx-markdown*.
</div>
`,
styles: []
})
export class AppComponent {}
Using markdown
Component:
Alternatively, you can use the markdown
component to load Markdown from a URL or variable:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<markdown [src]="'/assets/sample.md'"></markdown>
`,
styles: []
})
export class AppComponent {}
Loading External Markdown Files
To load an external Markdown file, place your .md
files in the assets
directory and reference them using the src
attribute:
<markdown src="/assets/sample.md"></markdown>
Ensure you have the Markdown file sample.md
in the src/assets
directory:
# Sample Markdown File
This is content loaded from an external Markdown file.
Syntax Highlighting for Code Blocks
ngx-markdown
integrates with highlight.js
to provide syntax highlighting for code blocks. To enable this, import highlight.js
styles in your angular.json
:
"styles": [
"node_modules/highlight.js/styles/github.css",
"src/styles.css"
],
Then, configure highlight.js
in AppModule
:
import { MarkdownModule, MarkedOptions, MarkdownService } from 'ngx-markdown';
@NgModule({
imports: [
BrowserModule,
MarkdownModule.forRoot({
markedOptions: {
provide: MarkedOptions,
useValue: {
gfm: true,
tables: true,
breaks: false,
pedantic: false,
sanitize: false,
smartLists: true,
smartypants: false,
},
},
loader: HttpClient,
})
],
})
export class AppModule {}
Conclusion
Integrating Markdown into your Angular application using ngx-markdown
provides a seamless and powerful way to manage and render content. With support for external files, custom configuration, and syntax highlighting, ngx-markdown
enriches the user experience and simplifies content formatting. Whether creating blogs, documentation, or any text-rich site, ngx-markdown
is a valuable addition to your Angular toolkit.
I hope this article helps you understand how to integrate and use ngx-markdown
in your Angular projects!
Comments ()