Markdown & Vue 3: Frontend Efficiency
Combining Markdown with Vue 3 enhances frontend development efficiency. Use vue-markdown-loader and marked to seamlessly integrate Markdown content into Vue 3 apps, enabling dynamic content management and display.
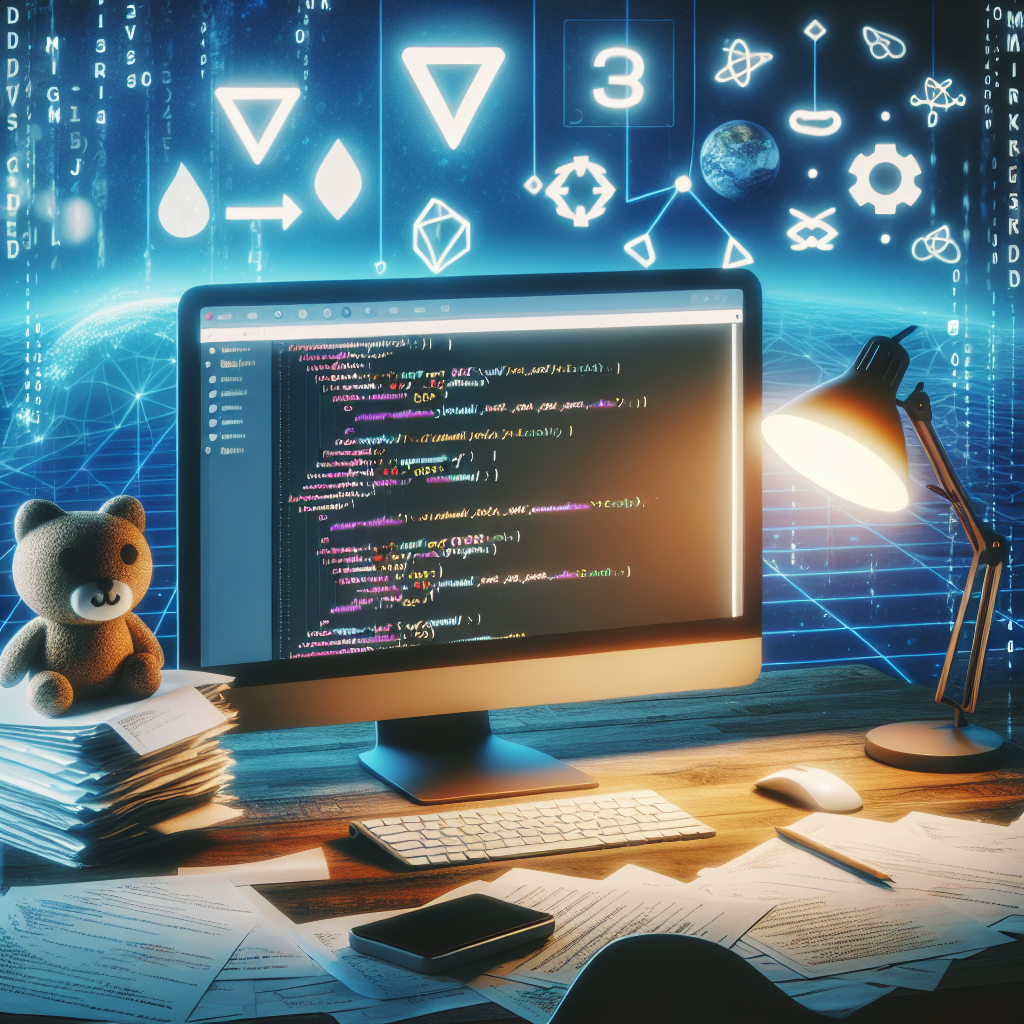
"Explore our suite of free Markdown toolsto convert, format, and enhance your documents with ease."
In the rapidly evolving field of frontend development, choosing the right tools and technology combinations is crucial for enhancing development efficiency and user experience. Markdown, as a lightweight markup language, has become the preferred tool for content creation and documentation due to its simple syntax and powerful functionality. Vue 3, the latest version of the Vue.js framework, brings faster performance and an improved development experience. This article will explore how to combine Markdown with Vue 3 to build efficient, dynamic frontend applications.
Why Choose Markdown?
The popularity of Markdown is no accident. It is easy to learn, quickly converts text into structured content, and is ideal for writing documents, blog posts, and README files. Markdown's syntax is intuitive, supporting elements such as links, images, and code blocks, and can be easily converted to HTML for display on web pages.
New Features of Vue 3
Vue 3 introduces the Composition API, a new way to organize and reuse logic, offering better logical composition and code organization compared to Vue 2's Options API. Additionally, Vue 3 has significantly improved performance by using Proxy instead of Object.defineProperty, making the reactive system more efficient.
Combining Markdown with Vue 3
Integrating Markdown into Vue 3 applications brings many benefits. It allows developers to write content in Markdown and dynamically render this content through Vue components. This makes content management more flexible while maintaining the separation of content and presentation.
Using vue-markdown-loader
vue-markdown-loader
is a Webpack loader that converts Markdown files into Vue components. With this tool, you can directly import Markdown files into Vue components and use them in templates.
// Install vue-markdown-loader
npm install vue-markdown-loader --save-dev
// Add loader in webpack configuration
module.exports = {
module: {
rules: [
{
test: /\.md$/,
loader: 'vue-markdown-loader'
}
]
}
}
Dynamically Rendering Markdown Content
In Vue 3, you can use libraries like marked
to parse Markdown content and render it into components.
<template>
<div v-html="markdownContent"></div>
</template>
<script>
import { ref, onMounted } from 'vue';
import marked from 'marked';
export default {
setup() {
const markdownContent = ref('');
onMounted(() => {
fetch('/path/to/markdown/file.md')
.then(response => response.text())
.then(text => {
markdownContent.value = marked(text);
});
});
return {
markdownContent
};
}
}
</script>
Conclusion
The combination of Markdown with Vue 3 provides frontend developers with an efficient and flexible way to manage and display content. By using tools like vue-markdown-loader
and marked
, developers can easily integrate Markdown content into Vue 3 applications, thereby enhancing development efficiency and user experience. Whether building documentation sites, blog platforms, or complex web applications, the combination of Markdown and Vue 3 demonstrates significant potential.
Comments ()