Svelte Markdown: Combining Svelte with Markdown for Dynamic Web Applications
Combining Svelte with Markdown enables content management and dynamic user interfaces. Use the marked library to parse Markdown and the @html directive for safe HTML rendering. Ideal for content-driven apps like blogs and documentation.
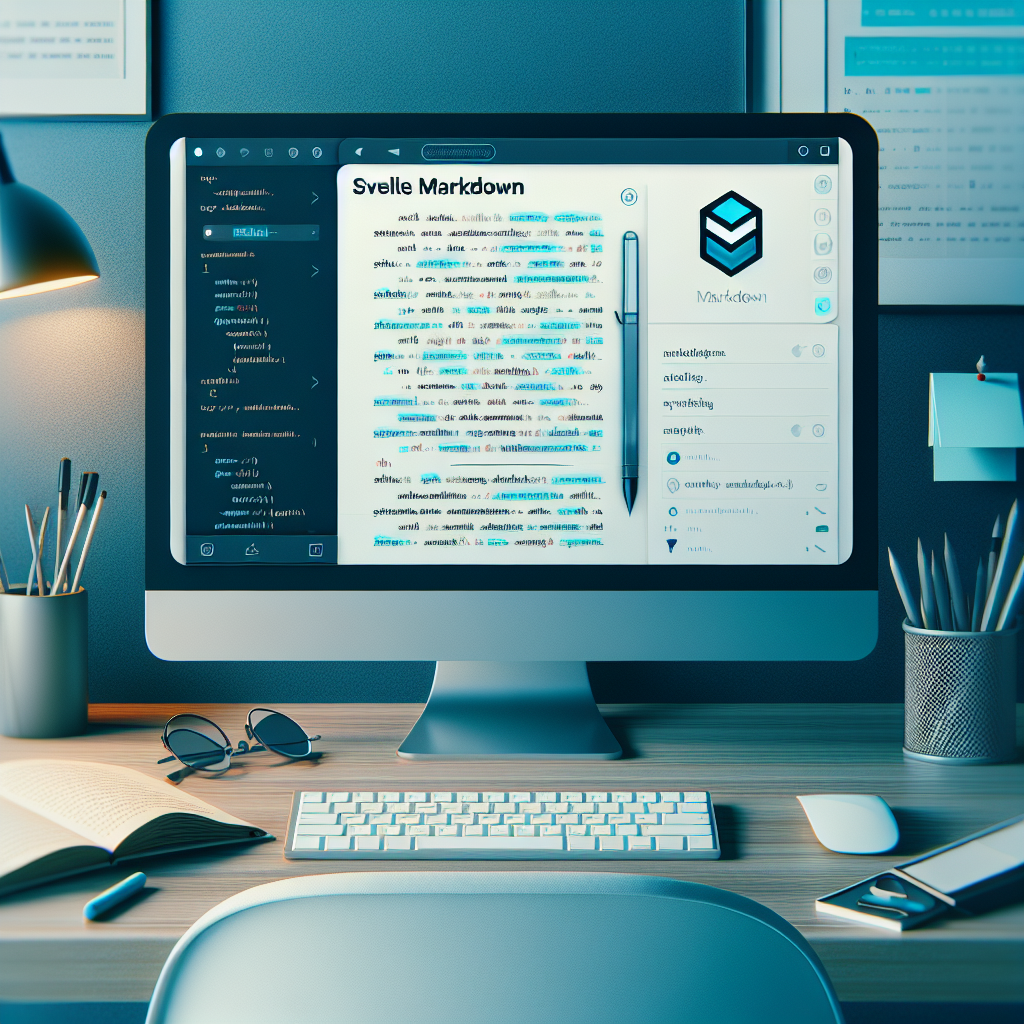
"Don't waste another minute formatting Markdown by hand. Try our free tools now and see the difference!"
Introduction
Svelte is a modern JavaScript framework for building user interfaces that compiles components to highly efficient vanilla JavaScript at build time. Markdown, a lightweight markup language, is widely used for formatting text. Combining Svelte with Markdown allows developers to create dynamic, content-rich web applications with ease. This article explores how to integrate and use Markdown within Svelte applications effectively.
Why Combine Svelte and Markdown?
- Content Management: Markdown simplifies the creation and management of content, making it ideal for blogs, documentation, and other content-heavy sites.
- Dynamic UIs: Svelte's reactive nature ensures that your web application remains dynamic and responsive, updating seamlessly as the underlying content changes.
- Ease of Use: Markdown is easy to write and read, providing a more intuitive way to manage content compared to HTML.
- SEO Benefits: Well-structured Markdown can enhance SEO, as it promotes the use of semantic HTML elements.
Setting Up Svelte with Markdown
To get started with Svelte and Markdown, you'll need a Svelte project. If you don't have one yet, you can create it using the following commands:
-
Initialize Svelte Project:
npx degit sveltejs/template svelte-markdown cd svelte-markdown npm install
-
Install Markdown Parsing Library: Next, you need a library for parsing Markdown.
marked
is a popular choice:npm install marked
Creating a Markdown Component
With the setup complete, let's create a Svelte component that takes Markdown content and renders it as HTML.
-
Markdown.svelte
:
<script> import { marked } from 'marked'; export let content = ''; </script> <div> {@html marked(content)} </div> <style> /* You can add styles for your markdown content here */ div { padding: 1rem; background: #f9f9f9; border-radius: 8px; } </style>
This component uses the @html
directive to render the converted HTML from the Markdown content safely.
Using the Markdown Component
You can now use the Markdown component in your Svelte application to display Markdown content dynamically.
-
App.svelte
:
<script> import Markdown from './Markdown.svelte'; let markdownContent = ` # Hello, Svelte! This is a **Markdown** component. - Item 1 - Item 2 - Item 3 [Svelte](https://svelte.dev) `; </script> <main> <h1>Welcome to Svelte Markdown</h1> <Markdown {content}={markdownContent} /> </main> <style> main { font-family: Arial, sans-serif; padding: 2rem; max-width: 800px; margin: auto; } </style>
This example imports the Markdown component, sets some sample Markdown content, and renders it within the main application layout.
Enhancing the Markdown Experience
To enhance the functionality further, you can add support for additional features such as:
-
Highlighting Code Blocks: Install a highlighting library like
highlight.js
and adapt theMarkdown.svelte
component.npm install highlight.js
-
Enhanced Markdown.svelte:
<script> import { marked } from 'marked'; import hljs from 'highlight.js'; import 'highlight.js/styles/default.css'; export let content = ''; marked.setOptions({ highlight: function (code, lang) { return hljs.highlightAuto(code, [lang]).value; } }); </script> <div> {@html marked(content)} </div>
-
Styling Enhancements: Add specific styles to better format the rendered Markdown content.
<style> div { padding: 1rem; background: #f9f9f9; border-radius: 8px; } h1 { color: #007acc; } p { line-height: 1.5; } pre { background: #282c34; color: #61dafb; padding: 1rem; border-radius: 8px; } code { font-family: 'Source Code Pro', monospace; } </style>
Conclusion
Integrating Markdown into Svelte applications provides a powerful combination of simplicity and dynamism, enabling developers to manage content efficiently while delivering responsive and engaging user interfaces. Whether building blogs, documentation sites, or content-heavy applications, leveraging Svelte with Markdown can significantly streamline development and enhance the user experience.
Comments ()