Using Markdown in Nuxt
Integrate Markdown with Nuxt.js using the @nuxt/content module. Store content in Markdown files, fetch and display them dynamically, and customize rendering for a powerful and flexible content solution.
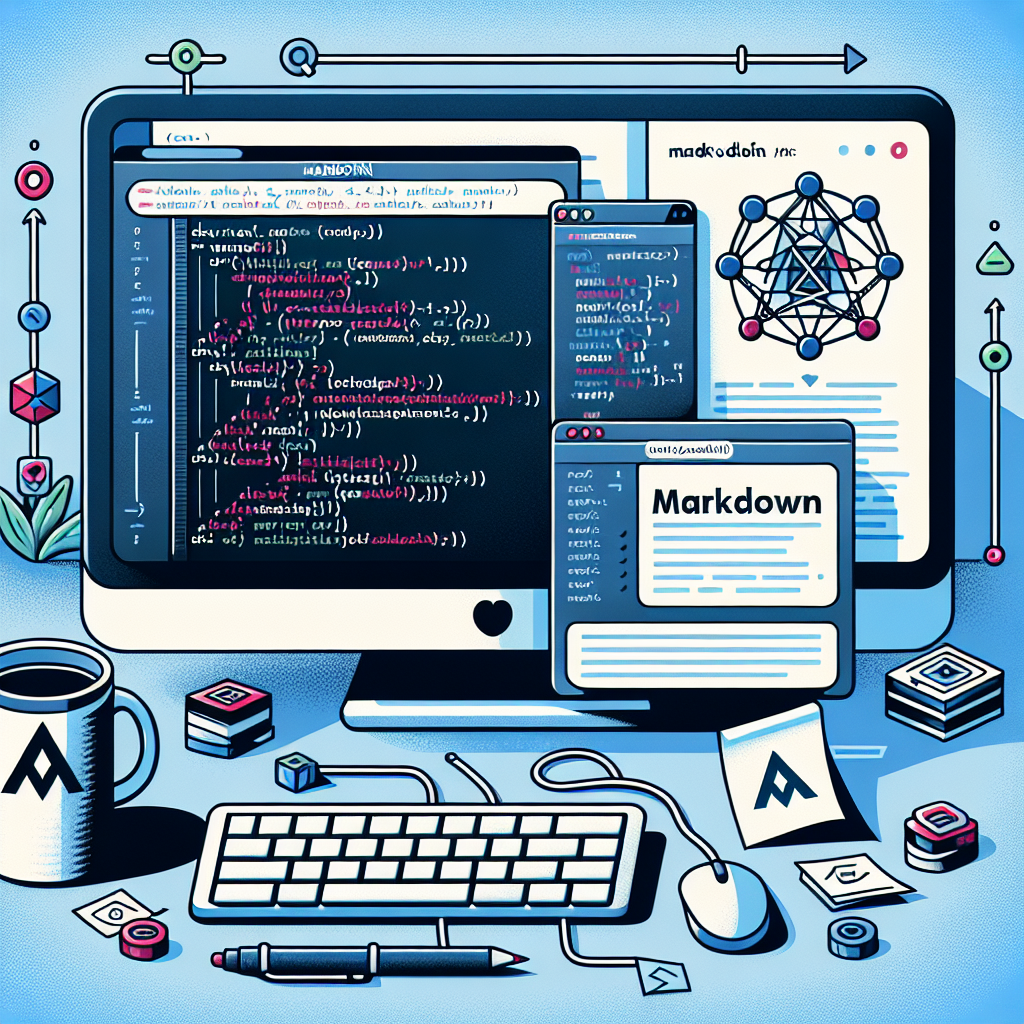
"Why struggle with Markdown formatting? Our free tools make it easy to create beautiful, professional-looking documents in seconds."
Introduction
Nuxt.js is a powerful framework for building server-rendered Vue.js applications. It provides a high-level abstraction that simplifies the development of Vue.js applications while offering powerful features for routing, code-splitting, and server-side rendering (SSR). One of the common requirements for content-heavy websites is the ability to write content in Markdown, a lightweight markup language. In this article, we will explore how to use Markdown in Nuxt.js.
What is Markdown?
Markdown is a plain-text formatting syntax that allows you to write content in a simple and readable format. It can be easily converted to HTML, making it ideal for content creation in web applications. Markdown is especially popular among developers due to its readability and ease of use.
Setting Up a Nuxt Project
First, let's create a new Nuxt.js project if you haven't already. If Nuxt CLI is not installed, you can install it globally with npm or yarn:
npm install -g create-nuxt-app
Create a new project:
npx create-nuxt-app my-nuxt-project
cd my-nuxt-project
Installing Markdown Dependencies
To handle Markdown files in a Nuxt.js project, you can use a combination of markdown-it and @nuxt/content. The @nuxt/content
module provides a powerful Markdown parser and integrates seamlessly with Nuxt. Let's install it:
npm install @nuxt/content
Then, add @nuxt/content
to the modules
array in your nuxt.config.js
:
export default {
modules: ['@nuxt/content'],
}
Creating Markdown Content
With the @nuxt/content
module installed, you can start adding Markdown files to your project. Create a content
directory in the root of your project and add your Markdown files there. For example, create content/blog/my-first-post.md
:
---
title: My First Post
description: Learning how to use Markdown in Nuxt
---
# Welcome to my blog
This is my first post written in Markdown!
Fetching Markdown Content
To fetch and display Markdown content in your Nuxt application, you can leverage the @nuxt/content
module's built-in methods. Create a new page in the pages
directory, for example pages/blog/_slug.vue
:
<template>
<article>
<h1>{{ post.title }}</h1>
<nuxt-content :document="post" />
</article>
</template>
<script>
export default {
async asyncData({ $content, params }) {
const post = await $content('blog', params.slug).fetch();
return { post };
}
}
</script>
<style>
article {
max-width: 700px;
margin: auto;
padding: 20px;
}
</style>
Dynamic Routing
Nuxt.js offers powerful dynamic routing out of the box. The pages/blog/_slug.vue
file automatically creates a dynamic route for each Markdown file in the content/blog
directory. For example, the content/blog/my-first-post.md
file can be accessed at the /blog/my-first-post
URL.
Customizing Markdown Rendering
The @nuxt/content
module uses markdown-it to parse Markdown files, allowing for customization of the Markdown rendering process. You can add plugins or customize the renderer in your nuxt.config.js
:
export default {
modules: ['@nuxt/content'],
content: {
markdown: {
prism: {
theme: 'prism-themes/themes/prism-material-oceanic.css'
},
remarkPlugins: ['remark-math'],
rehypePlugins: ['rehype-katex']
}
}
}
Conclusion
Using Markdown in Nuxt.js is a powerful way to manage content in a simple, readable format. The @nuxt/content
module provides seamless integration with Nuxt, offering features like dynamic routing and easy content fetching. With the ability to customize Markdown parsing, you can tailor the experience to fit your needs, making Nuxt and Markdown a perfect combination for content-rich applications.
I hope this article helps you understand how to use Markdown in your Nuxt.js projects!
Comments ()