Using Pandas to Convert DataFrames to Markdown
Pandas offers the to_markdown() method to easily convert DataFrames into Markdown tables. It supports customizing output, such as adjusting alignment and disabling index. The result can be saved as a .md file, ideal for dynamic reports and documentation.
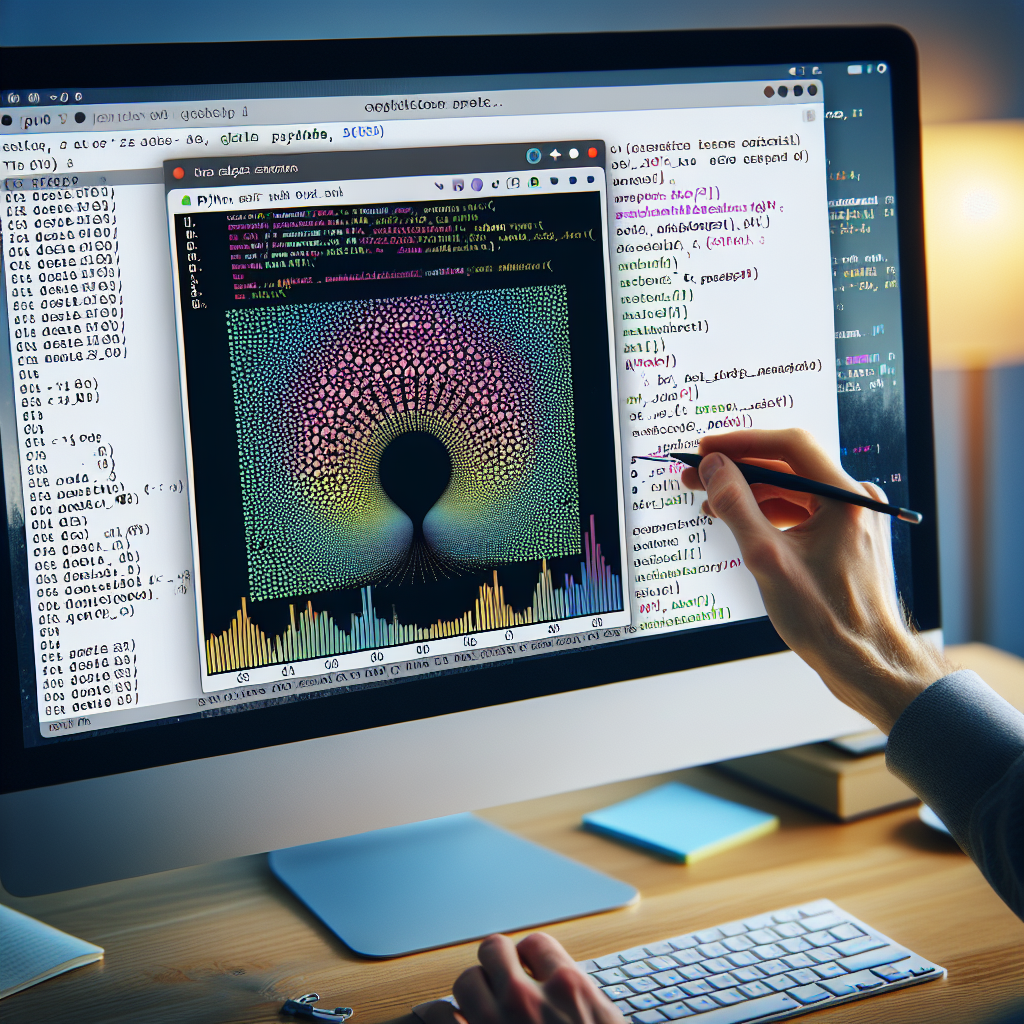
"Why struggle with Markdown formatting? Our free tools make it easy to create beautiful, professional-looking documents in seconds."
Introduction
Pandas is a powerful Python library widely used for data manipulation and analysis. One of its lesser-known but highly useful features is the ability to convert DataFrames into various formats, including Markdown. This capability is particularly valuable for integrating data previews into markdown documents, which are commonly used for documentation, reports, and static site generators like Jekyll and Hugo. In this article, we will explore how to convert Pandas DataFrames to Markdown format efficiently.
Setting Up Your Environment
Before we start, ensure you have Pandas installed. If not, you can install it using pip:
pip install pandas
Additionally, make sure you have a basic understanding of how to create and manipulate DataFrames in Pandas.
Creating a DataFrame
To demonstrate the conversion process, let's first create a simple DataFrame:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
print(df)
This will output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Converting DataFrame to Markdown
Pandas provides a straightforward method, to_markdown()
, for converting a DataFrame to a Markdown-formatted string. Here’s how you can use it:
markdown = df.to_markdown()
print(markdown)
This will produce the following Markdown table:
| | Name | Age | City |
|---:|:--------|------:|:------------|
| 0 | Alice | 25 | New York |
| 1 | Bob | 30 | Los Angeles |
| 2 | Charlie | 35 | Chicago |
Customizing the Markdown Output
Formatting Options
The to_markdown()
method includes several parameters for customizing the output. For example, you can adjust the table's alignment or disable indexing:
markdown = df.to_markdown(index=False)
print(markdown)
Output without row indices:
| Name | Age | City |
|:--------|------:|:------------|
| Alice | 25 | New York |
| Bob | 30 | Los Angeles |
| Charlie | 35 | Chicago |
Column Formatting
You can also format specific columns before converting them to Markdown. For instance, if you want to convert the 'Age' column to a string with a 'years' suffix:
df['Age'] = df['Age'].astype(str) + ' years'
markdown = df.to_markdown(index=False)
print(markdown)
This will result in:
| Name | Age | City |
|:--------|:-----------|:------------|
| Alice | 25 years | New York |
| Bob | 30 years | Los Angeles |
| Charlie | 35 years | Chicago |
Saving the Markdown to a File
To save the Markdown table to a file, you can simply write the string to a file with a .md
extension:
with open("data_table.md", "w") as file:
file.write(markdown)
This will create a file named data_table.md
with your DataFrame as a Markdown table.
Conclusion
Converting Pandas DataFrames to Markdown is a convenient feature for integrating data into markdown-based documents. This can be particularly useful for creating dynamic reports, documentation, or static sites. With Pandas' to_markdown()
method, you can easily customize and save your DataFrames in a readable and shareable format. Whether you're a data scientist, developer, or content creator, this skill can enhance the way you present and distribute data effectively.
Comments ()